NodeJS – Updating to the Latest Minor Version
Estimated reading time: 3 minutes
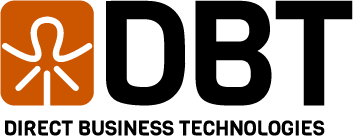
Welcome!
Welcome to our blog! Today I am sharing out some simple Bash scripts that will update NodeJS to the latest minor version. I wrote these scripts to be utilized with our patch platform, Automox. Within the Automox platform, there are two types of scripts that are run; First we have the evaluation code, this checks to determine if the system is in compliance. Second, we have the remediation code, this gets executed when the device is not in compliance. Our scripts below are broken out to accommodate this overall process, you likely may want to combine these scripts for your own use if you do not use Automox.
We use these scripts on various Secret Double Octopus instances, but these will work on other web and application servers. Secret Double Octopus supports upgrading to the latest minor version, they do not support changing major versions of NodeJS.
Node Version Manager (NVM)
First, we need to make sure NVM is installed on your system. NVM is short for Node Verson Manager and is a project designed to help you manage NodeJS installations. Details can be found at the GitHub repo https://github.com/nvm-sh/nvm. We have evaluation code that checks to see if NVM is present. If it is present, then the script exits with status code 0, this indicates the device is in compliance with the Automox worklet/policy. If NVM is not present, the script will exit with status code 1, indicating the device is not compliant. For devices that are not compliant, the remediation code will be run on the day/time specified in the Automox policies.
Disclaimer: All scripts and code presented on this blog are use at your own risk. It is up to you to test this code in a controlled environment. This code works for us, tweaks may be needed for your systems.
NVM Evaluation code:
#!/bin/bash
# Try to load NVM
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
# Check if NVM is installed by checking if nvm command is available
if ! command -v nvm &> /dev/null
then
echo "NVM is not installed."
exit 1 # NVM is not installed, update needed
else
echo "NVM is installed."
exit 0 # NVM is installed, no update needed
fi
NVM Remediation Code:
#!/bin/bash
# Function to check if NVM is installed
check_nvm_installed() {
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
# Check if the nvm command is available
command -v nvm &> /dev/null
}
# Install NVM if it's not installed
if ! check_nvm_installed
then
echo "Installing NVM..."
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.7/install.sh | bash
# Check if NVM was successfully installed
if check_nvm_installed
then
echo "NVM installation successful."
else
echo "NVM installation failed."
exit 1 # Exit with error if installation fails
fi
else
echo "NVM is already installed."
fi
NodeJS Update via NVM
Now that we have NVM installed, we need to check for the version of NodeJS and whether or not an update is needed. Just like with NVM, we have our Automox environment configured to check to see if the current version of NodeJS is at the latest minor version. If it is, then the evaluation code will exit with status code 0, if it is not, then the evaluation code will exit with status code 1.
NodeJS Evaluation Code:
#!/bin/bash
# Load nvm
source ~/.nvm/nvm.sh
# Get current Node version
current_version=$(node -v)
current_version=${current_version:1} # Remove the 'v' prefix
# Extract major and minor version
base_version=$(echo $current_version | cut -d. -f1,2)
# Get the latest LTS version in the same family
latest_version=$(nvm ls-remote "v$base_version.*" | grep -oP 'v\d+\.\d+\.\d+' | tail -1)
# Check if the latest version is different from the current version
if [ "$latest_version" != "v$current_version" ]; then
echo "Update Needed"
exit 1 # Update needed
else
echo "No Update Needed"
exit 0 # No update needed
fi
NodeJS Remediation Code:
#!/bin/bash
# Load nvm
source ~/.nvm/nvm.sh
# Get current Node version
current_version=$(node -v)
current_version=${current_version:1} # Remove the 'v' prefix
# Extract major and minor version
base_version=$(echo $current_version | cut -d. -f1,2)
# Install the latest LTS version in the same family
nvm install "$base_version"
That’s All Folks
Thanks for taking a couple of minutes of your day to read! I hope you have found this useful. Have a great day and week ahead! https://www.dbtsupport.com/about-us/blog/. Check back again for new content!